Introduction
On this page, you'll learn the basics of Python, one of the most popular computer programming languages in the world.
This is NOT a comprehensive guide to all Python documentation. For a more details, see the official Python 3.12.3 Documentation
Why learn Python?
Python has many real-world applications, including:
- Building apps
- Analyzing and plotting data
- Machine learning and AI
- Web development
In addition, Python code is easy to read, making it a great programming language for beginner coders to learn.
Install Python
Before using Python, you need to install it to your computer.
If you don't want to install Python, check out Jupyter Notebooks, a great web-based code editor that allows you to run Python without installing it.
1. Hello world
This tutorial is based how Python is run in the built-in Mac terminal.
For our first lesson, let's write a program that prints "Hello World!" To start, open your terminal, type python3 -i
to start an interactive Python session.
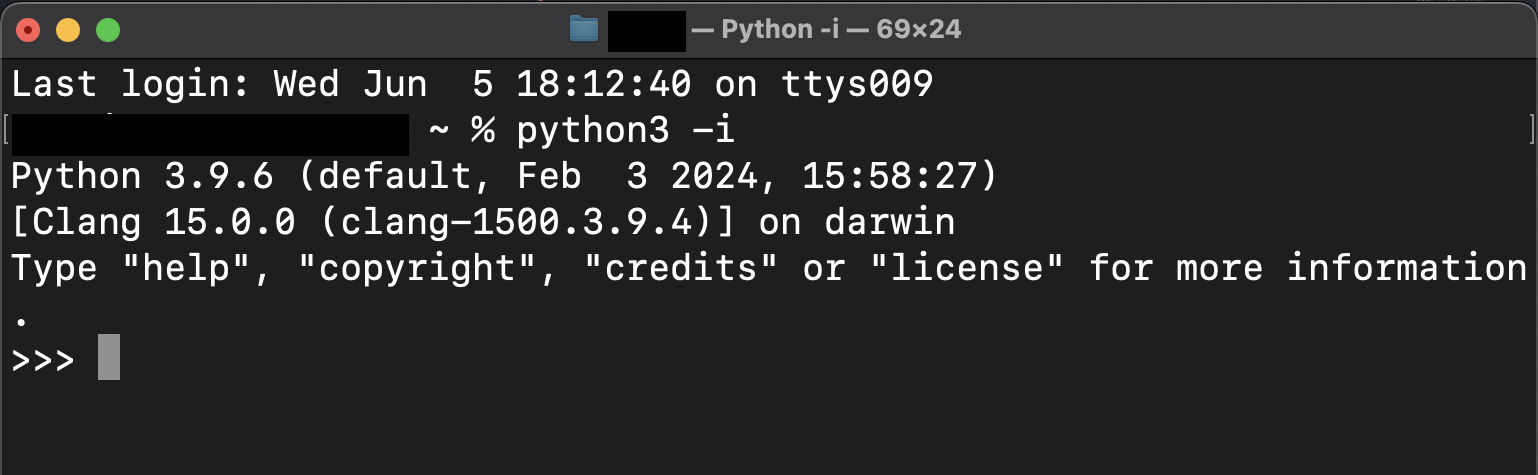
Now type in the following code: print("Hello world!")
and hit Enter. The print()
function prints the text inside quotation marks.

2. Basic Arithmetic
If you type a number and press Enter, Python will return that number.
>>> 2
2
If you give it an expression, Python will return the result of computing that expression.
>>> 5 * 3
15
3. Variables
One of the most powerful features of Python is the ability to create and manipulate variables. For example, let's create a variable called x. Then, let's do some arithmetic on x.
>>> x = 5
>>> x + 3
8
As you can see, Python stores the value 5 under the name x.
There are many data types you can set a variable to: ints, floats, Booleans (True or False), and strings.
4. If-statements
If-statements tell your code to run only under certain circumstances.
>>> if x > 3:
... print("Hello world")
>>> else :
>>> ... print("Bananas")
Hello world
If-statement syntax:
if [condition]:
[code]
elif [condition]:
[code]
else:
[code]
Elif, which stands for else-if, tells Python what code to run if the first if condition is False.
Python will check the boolean conditions (also known as "clauses") like so:
- Check if if condition is true
- If the if condition is true, run that code block and skip all the other elifs and the else clause.
- If the if condition is false, check the elif condition.
5. For & While loops
A great way to make code run multiple times without having to copy-and-paste your code over and over is to use a for loop or a while loop.
A for loop allows you to repeat code either (a) a certain number of times or (b) across a sequence (e.g. list, tuple, etc.).
The example below assigns x = 1, then x = 2, then x = 3, running the indented code block each time.
for x in [1, 2, 3]:
print(x)
1
2
3
A while loop also repeats code, but only when a condition is met.
x = 3
while x < 5:
print(x)
x = x + 1 # each time the while loop runs, x increases by 1
3
4